Arduino
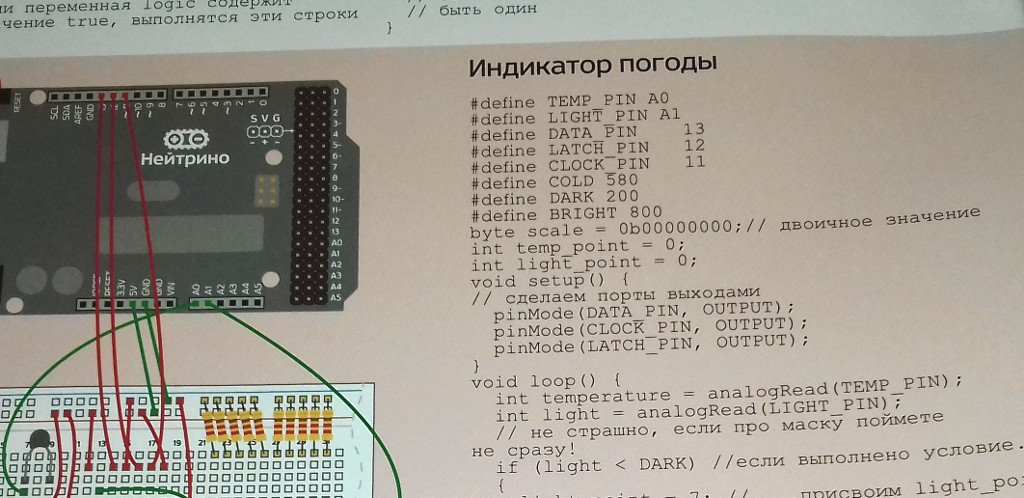
We continue publishing the online course “Building robots and other devices on Arduino”, starting here.
So, for a long time, the second week of the online course of robotics from MIPT ended. To admit, the week turned out to be very full of all sorts of topics.
Here is an indicative list, which I singled out for myself:
- Voltage Divider. Use of photoresistor and thermistor
- Analog signal. The bit depth of the signal
- Communication through the serial port. Processing Environment
- A digital signal. Buttons and connection options. The pull-up resistor
- Logical expressions, if and else statements
- Buzzer, LED scale, seven-segment indicator
- Microcircuits. Logical inverter 74HC04, shift register 74HC595
- Debugging programs
- External Modules
- A variant of a ready-made monitoring system that displays the temperature and the level of illumination on an LED scale, as well as a loudspeaker triggered when a certain temperature is exceeded
Having successfully passed the test and collected the proposed schemes from the lessons, I thought about how to improve this or that scheme or to collect something of my own.
The first thing that came to mind – Upgrading of the light sensor. The implementation proposed in the lesson simply removed the value from the photoresistor and sent it to the serial port.
The upgraded version should use a seven-segment indicator to output numbers from 0 (minimum illumination) to 9 (maximum illumination). The indicator must be connected via a shift register. Use the two buttons to set the minimum and maximum light levels. The shift register is needed in order not to use Arduino on each segment, but instead use a smaller number of pins. In fact, the shift register converts the serial data output (one bit per time unit) into a parallel (several bits per unit of time). In our case, instead of seven Arduino pins, we only need three.
In the Fritzing editor, I got such a device.
So it looks alive.
]
Note that the photoconductor is connected in a slightly different way on the PCB than in the video lesson – where we shot the value of the voltage on the photoresistor relative to the ground, and in the circuit we take the voltage drop with respect to Power supply. This is done to simplify the program a little – as the illumination level increases, the photoresistor resistance decreases. Consequently, at the same current, the voltage drop is reduced. Therefore, on the analog input, the higher the voltage, the higher the illumination level, and vice versa.
Now it’s up to small – to refine the source code. The program was based on the same program for deriving the value for the seven-segment indicator.
// Pins required for the shift register operation
#define DATA_PIN 13
#define LATCH_PIN 12
#define CLOCK_PIN 11
// The pins of the buttons responsible for setting the minimum and maximum values
#define BTN_MIN 3
#define BTN_MAX 2
// Pin, from which we will take the values of the photoresistor
#define SENS_PIN A5
// The values of the register outputs for the indicator, corresponding to the digits
Byte d0 = 0b01111101;
Byte d1 = 0b00100100;
Byte d2 = 0b01111010;
Byte d3 = 0b01110110;
Byte d4 = 0b00100111;
Byte d5 = 0b01010111;
Byte d6 = 0b01011111;
Byte d7 = 0b01100100;
Byte d8 = 0b01111111;
Byte d9 = 0b01110111;
// Predefined minimum and maximum light levels
Int min_light = 0;
Int max_light = 1023;
// The current value of the sensor
Int value;
// Value limited to min. And max. The level of
Int output;
// Output digit
Int digit;
Void setup ()
{
// Set the shift register pins
PinMode (DATA_PIN, OUTPUT);
PinMode (CLOCK_PIN, OUTPUT);
PinMode (LATCH_PIN, OUTPUT);
// Turn on the port for debugging information
Serial.begin (9600);
// Set the pins for the buttons
PinMode (BTN_MIN, INPUT_PULLUP);
PinMode (BTN_MAX, INPUT_PULLUP);
}
Void loop ()
{
// Get the value from the photoresistor
Value = analogRead (SENS_PIN);
Output = value;
// If buttons are pressed – set threshold values
If (!, digitalRead (BTN_MIN)) min_light = value;
If (! DigitalRead (BTN_MAX)) max_light = value – 10;
// Apply top and bottom constraints
If (value < min_light) output = min_light; if (value > max_light) output = max_light;
// Get the digit that should be output to the indicator
Digit = map (value, min_light, max_light, 0, 9);
// Debug information
Serial.println (“Value:” + String (value) + “Output:” + String (output) + “Min:” + String (min_light) + “Max:” + String (max_light) + “Current:” + String (Value) + “Digit:” + String (digit));
// Output the digit directly to the indicator
If (digit == 0)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d0);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 1)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d1);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 2)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d2);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 3)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d3);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 4)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d4);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 5)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d5);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 6)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d6);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 7)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d7);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 8)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d8);
DigitalWrite (LATCH_PIN, HIGH);
}
Else if (digit == 9)
{
DigitalWrite (LATCH_PIN, LOW);
ShiftOut (DATA_PIN, CLOCK_PIN, LSBFIRST, d9);
DigitalWrite (LATCH_PIN, HIGH);
}
// Delay for smoother output
Delay (10);
From the features – when setting the maximum level of illumination, we had to subtract a certain constant (max_light = value – 10), selected empirically. This is necessary in order to avoid “rattling” at the maximum level of illumination, since the value of the voltage taken from the photoresistor is unstable.
We compile the sketch, load it into Arduino and check it.
The monitor of the port …
And then live
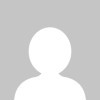